Anna Blog C+: Your Ultimate Guide to C+ Programming
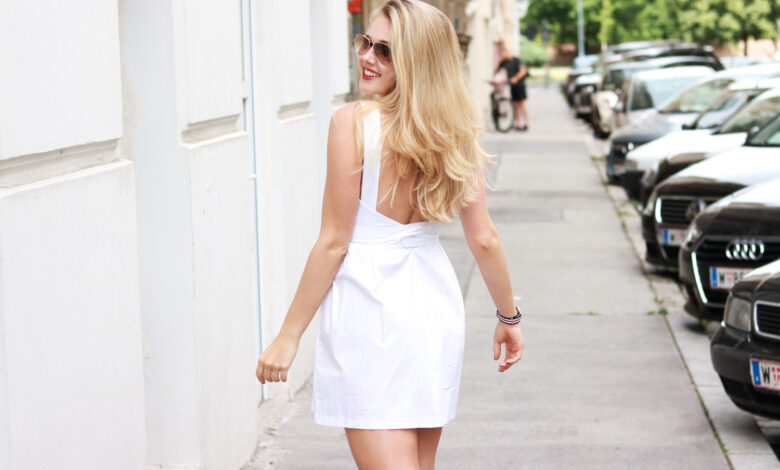
Welcome to Anna Blog C+, your go-to resource for all things related to C+ programming. Whether you’re a beginner just starting out or an advanced developer looking to refine your skills, this guide has something for you. We’ll cover a wide range of topics, from basic programming tips to advanced techniques, best practices, and more. Let’s dive in!
Anna Blog C+ Programming Tips for Beginners
Understanding the Basics
Starting with C+ can be daunting, but mastering the basics is Anna Blog C+ is an extension of the C language, incorporating object-oriented features and providing more flexibility. Begin with understanding fundamental concepts like variables, data types, and control structures (loops, if-else statements).
Variables and Data Types
Variables are the building blocks of any program. Anna Blog C+, you’ll work with various data types such as int, float, char, and double. Each data type serves a different purpose and allows you to store different kinds of information. For instance, ‘int’ is used for integers, while ‘char’ is used for characters.
Control Structures
Control structures guide the flow of your program. Loops like ‘for’ and ‘while’ help in iterating over a block of code multiple times. Conditional statements like ‘if-else’ allow your program to make decisions based on certain conditions. Mastering these basics will set a solid foundation for more complex topics Anna Blog C+.
Setting Up Your Development Environment
To start codingAnna Blog C+ you’ll need a proper development environment. There are various IDEs (Integrated Development Environments) like Visual Studio, Code::Blocks, and Eclipse. These tools offer features like syntax highlighting, debugging, and auto-completion, which make coding easier Anna Blog C+.
Choosing the Right IDE
Selecting the right Anna Blog C+ depends on your needs. Visual Studio is popular for its comprehensive features, but it might be overwhelming for beginners. Code::Blocks is simpler and more intuitive, making it a great choice if you’re just starting out.
Installing and Configuring Your IDE
Once you’ve chosen your IDE, follow the installation instructions specific to your operating system. Configure your IDE by setting up the compiler and linking necessary libraries. This step ensures that your programs compile and run smoothly Anna Blog C+.
Anna Blog C+ Advanced Techniques and Tricks
Memory Management
Understanding memory management is crucial for advanced C+ programming. C+ gives you direct control over memory allocation and deallocation, which is both powerful and risky. Mismanagement can lead to issues like memory leaks and segmentation faults Anna Blog C+.
Dynamic Memory Allocation
Dynamic memory allocation involves using pointers and functions like malloc() and free() to allocate and deallocate memory during runtime. This technique is essential for handling large data sets or when the size of the data structure isn’t known at compile time.
Smart Pointers
To simplify memory management, C+11 introduced smart pointers. Smart pointers like std::unique_ptr and std::shared_ptr automate memory management, reducing the risk of memory leaks and dangling pointers. Understanding and utilizing smart pointers can greatly improve your code’s safety and efficiency.
Templates and Generics
Templates are a powerful feature in C+ that allow you to write generic and reusable code. They enable functions and classes to operate with any data type, reducing code duplication and increasing flexibility.
Function Templates
Function templates allow you to create a single function that works with different data types. For example, you can write a generic sort function that sorts arrays of integers, floats, or any other data type using the same code.
Class Templates
Class templates extend the concept of templates to classes. They enable you to create data structures like linked lists, stacks, and queues that work with any data type. Mastering templates can significantly enhance your coding efficiency and codebase maintainability.
Anna Blog C+ Best Practices for Developers
Writing Clean and Readable Code
Clean and readable code is easier to understand, maintain, and debug. Following best practices in coding style can make a huge difference in the long run.
Naming Conventions
Use meaningful and descriptive names for variables, functions, and classes. Stick to a consistent naming convention throughout your codebase. For example, use camelCase for variables and functions, and PascalCase for class names.
Code Formatting
Proper indentation and spacing make your code more readable. Follow a consistent style guide and use tools like clang-format to automatically format your code. This practice helps maintain a uniform code style across your project.
Commenting and Documentation
Comments and documentation are crucial for understanding and maintaining your code. Write clear and concise comments explaining the purpose of complex code sections. Additionally, document your functions and classes using tools like Doxygen to generate comprehensive documentation.
Inline Comments
Inline comments explain specific lines or blocks of code. Use them sparingly and only when necessary. Over-commenting can clutter your code and make it harder to read.
Documentation Comments
Documentation comments provide detailed descriptions of functions, classes, and modules. These comments should describe the purpose, parameters, return values, and any exceptions thrown by the function or class.
Anna Blog C+ Common Mistakes to Avoid
Memory Leaks and Dangling Pointers
One of the most common pitfalls in C+ programming is improper memory management. Memory leaks occur when allocated memory is not properly deallocated, leading to wasted memory resources. Dangling pointers, on the other hand, point to memory that has already been freed, leading to undefined behavior.
Detecting and Fixing Memory Leaks
Use tools like Valgrind to detect memory leaks in your program. Regularly run your code through these tools to identify and fix memory management issues. Ensure that every is paired with a corresponding free() or delete.
Avoiding Dangling Pointers
To avoid dangling pointers, set pointers to nullptr after freeing the memory they point to. This practice helps in easily identifying and preventing the use of invalid pointers.
Off-by-One Errors
Off-by-one errors are common in loops and array indexing. These errors occur when the loop iterates one time too many or too few, leading to incorrect results or runtime errors.
Correct Loop Boundaries
Pay attention to loop boundaries and conditions. Ensure that loops start and end at the correct indices. Using < instead of <= (or vice versa) can often be the source of off-by-one errors.
Array Indexing
Always check array indices to ensure they are within valid bounds. Using out-of-bounds indices can lead to undefined behavior and program crashes.
Anna Blog C+ Step-by-Step Coding Tutorials
Building a Simple Calculator
Let’s start with a simple project: building a calculator. This project will help you understand basic concepts and syntax in C+.
Setting Up
Create a new project in your IDE and set up the necessary files. Begin by writing the main function and setting up a basic menu for the calculator.
Implementing Basic Operations
Implement functions for basic operations like addition, subtraction, multiplication, and division. Each function should take two operands as input and return the result.
Handling User Input
Write code to handle user input and call the appropriate functions based on the user’s choice. Ensure that the program handles invalid inputs gracefully.
Creating a To-Do List Application
Next, let’s build a more complex project: a to-do list application. This project will introduce you to data structures and file handling in C+.
Designing the Data Structure
Design a class to represent a to-do item. The class should have attributes like task description, due date, and priority. Implement methods to add, remove, and display tasks.
Implementing File Handling
Add functionality to save and load the to-do list from a file. This feature allows users to persist their tasks between sessions.
Adding User Interface
Create a simple text-based user interface for interacting with the to-do list. Implement menu options for adding, removing, and viewing tasks.
Anna Blog C+ Essential Libraries and Tools
Standard Template Library (STL)
The Standard Template Library (STL) is a powerful library in C+ that provides a collection of useful data structures and algorithms. It includes containers like vector, list, and map, as well as algorithms for sorting, searching, and manipulating data.
Using Containers
Containers in STL are data structures that store collections of objects. The vector container, for example, is a dynamic array that can resize itself automatically. The map container stores key-value pairs, allowing for efficient data retrieval based on keys.
Leveraging Algorithms
STL algorithms provide a range of functionalities, from sorting and searching to modifying data. Functions like can greatly simplify your code and improve its efficiency.
Boost Libraries
Boost is a set of libraries that extends the functionality of C+. It includes libraries for tasks like string manipulation, file handling, and multi-threading. Using Boost can save you time and effort by providing ready-made solutions for common programming problems.
String Manipulation
Boost provides powerful tools for string manipulation, such as for converting strings to uppercase and for splitting strings based on delimiters.
File Handling
Boost’s file handling libraries simplify tasks like reading from and writing to files. The library provides a comprehensive set of tools for working with file systems and directories.
Anna Blog C+ Debugging and Troubleshooting Guide
Common Debugging Techniques
Debugging is an essential skill for any programmer. Effective debugging can save you hours of frustration and help you quickly identify and fix issues in your code.
Using Debuggers
A debugger is a tool that allows you to step through your code, inspect variables, and set breakpoints. Tools like GDB and the built-in debuggers in IDEs like Visual Studio can help you find and fix bugs efficiently.